在IP地址规划中,涉及到计算大量的IP地址,包括网段、网络掩码、广播地址、子网数、IP类型等,别担心,Ipy模块拯救你。Ipy模块可以很好的辅助我们高效的完成IP的规划工作。
[root@localhost ~]# wget -c https://pypi.python.org/packages/source/I/IPy/IPy-0.81.tar.gz --no-check-certificate
[root@localhost ~]# tar zxf IPy-0.81.tar.gz
[root@localhost ~]# cd IPy-0.81
[root@localhost IPy-0.81]# python setup.py install
copying build/lib/IPy.py -> /usr/lib/python2.7/site-packages
byte-compiling /usr/lib/python2.7/site-packages/IPy.py to IPy.pyc
Writing /usr/lib/python2.7/site-packages/IPy-0.81-py2.7.egg-info
IPy模块包含IP类,使用它可以方便处理绝大部分格式为IPv6的网络和地址
Python 2.7.5 (default, Jul 13 2018, 13:06:57)
[GCC 4.8.5 20150623 (Red Hat 4.8.5-28)] on linux2
Type "help", "copyright", "credits" or "license" for more information.
>>> IPy.IP('10.0.0.0/8').version()
[root@aliyun_server_v2 ~]# cat ip.py
ip = IPy.IP('192.168.31.0/24')
[root@aliyun_server_v2 ~]# python ip.py
>>> ip.reverseNames() # 反向解析地址格式
['253.31.168.192.in-addr.arpa.']
>>> ip.iptype() # 私网类型
'PRIVATE'
>>> IP('8.8.8.8').iptype() # 公网类型
'PUBLIC'
>>> IP('8.8.8.8').int() # 转换为整型格式
134744072
>>> IP('8.8.8.8').strHex() # 转换为十六进制格式
'0x8080808'
>>> IP('8.8.8.8').strBin() # 转换成二进制格式
'00001000000010000000100000001000'
>>> print IP('0x8080808') # 十六进制转换为IP格式
8.8.8.8
>>> print IP(134744072) # 整型格式转换为IP格式
8.8.8.8
IP方法也支持网络地址的转换,例如根据IP和掩码产生网段格式
>>> print (IP('192.168.31.0').make_net('255.255.255.0'))
192.168.31.0/24
>>> print (IP('192.168.31.0/255.255.255.0',make_net=True))
192.168.31.0/24
>>> print (IP('192.168.31.0-192.168.31.255',make_net=True))
通过strNormal方法指定不同wantprefixlen参数值以定制不同输出类型的网段,输出类型为字符串
>>> IP('192.168.31.0/24').strNormal(0)
>>> IP('192.168.31.0/24').strNormal(1)
'192.168.31.0/24'
>>> IP('192.168.31.0/24').strNormal(2)
'192.168.31.0/255.255.255.0'
>>> IP('192.168.31.0/24').strNormal(3)
'192.168.31.0-192.168.31.255'
wantprefixlen = 0,无返回,如192.168.31.0
wantprefixlen = 1,prefix格式,如192.168.31.0/24
wantprefixlen = 2,ecimalnetmask格式,如192.168.31.0/255.255.255.0
wantprefixlen = 3,lastIP格式,如192.168.31.0-192.168.31.255
比较两个网段是否存在包含、重叠等关系,比如同网络但不同prefixlen会认为是不相等的网段,如10.0.0.0/16不等于10.0.0.0/24,另外即使具有相同的prefixlen但处于不同的网络地址,同样也视为不相等,如10.0.0.0/16不等于192.0.0.0/16。IPy支持类似于数值型数据的比较,以帮助IP对象进行比较。
>>> IP('10.0.0.0/24') < IP('12.0.0.0/24')
True
>>> '192.168.1.100' in IP('192.168.1.0/24')
True
>>> IP('192.168.1.0/24') in IP('192.168.0.0/16')
True
>>> IP('192.168.0.0/23').overlaps('192.168.1.0/24')
1 #返回1代表存在重叠
>> IP('192.168.1.0/24').overlaps('192.168.2.0/24')
0 #返回0代表不存在重叠
3)根据输入的IP或子网返回网络、掩码、广播、反向解析、子网数、IP类型等信息。
[root@localhost ~]# cat simple.py
#!/usr/bin/python
#coding=utf-8
from IPy import IP
#接受用户输入,参数为IP地址或网段地址
ip_s = raw_input('Please input an IP or net-range: ')
ips = IP(ip_s)
#为一个网络地址
if len(ips) > 1:
#输出网络地址
print('net: %s' % ips.net())
#输出网络掩码地址
print('netmask: %s' % ips.netmask())
#输出网络广播地址
print('broadcast: %s' % ips.broadcast())
#输出地址反向解析
print('reverse address: %s' % ips.reverseNames()[0])
#输出网络子网数
print('subnet: %s' % len(ips))
#为单个IP地址
else:
#输出IP方向解析
print('reverse address: %s' % ips.reverseNames()[0])
#输出十六进制地址
print('hexadecimal: %s' % ips.strHex())
#输出二进制地址
print('binary: %s' % ips.strBin())
#输出地址类型,如PRIVATE、PUBLIC、LOOPBACK等
print('iptype: %s' % ips.iptype())
Please input an IP or net-range: 192.168.31.0/24
net: 192.168.31.0
netmask: 255.255.255.0
broadcast: 192.168.31.255
reverse address: 31.168.192.in-addr.arpa.
subnet: 256
hexadecimal: 0xc0a81f00
binary: 11000000101010000001111100000000
iptype: PRIVATE
[root@localhost ~]# python simple.py
Please input an IP or net-range: 192.168.31.253
reverse address: 253.31.168.192.in-addr.arpa.
hexadecimal: 0xc0a81ffd
binary: 11000000101010000001111111111101
iptype: PRIVATE
若文章图片、下载链接等信息出错,请在评论区留言反馈,博主将第一时间更新!如本文“对您有用”,欢迎随意打赏,谢谢!
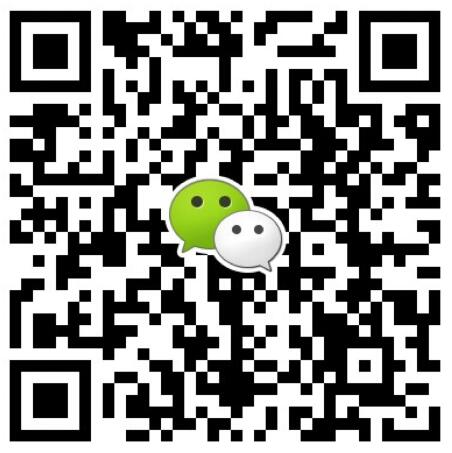
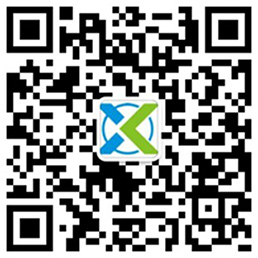
亲测,写的不错,感谢博主